Add Watermark
Use the Wordize Watermark for .NET module to add a watermark to your document.
Select any document conversion module to work with the required formats.
Wordize provides the Wordize Watermark module for .NET, which offers robust functionality for adding watermarks to your documents, enhancing security and branding. You can insert both text and image watermarks with customizable options to suit your requirements.
Watermark Types
A watermark is inserted behind the main document content. You can add an image or text watermark:
- Image watermark: inserts images, such as logos or seals, as watermarks
- Text watermark: inserts text such as “Confidential” or “Draft” as a watermark
Adding an Image Watermark
The functionality of adding an image watermark in Wordize is available through the Watermarker class, which provides SetImage methods.
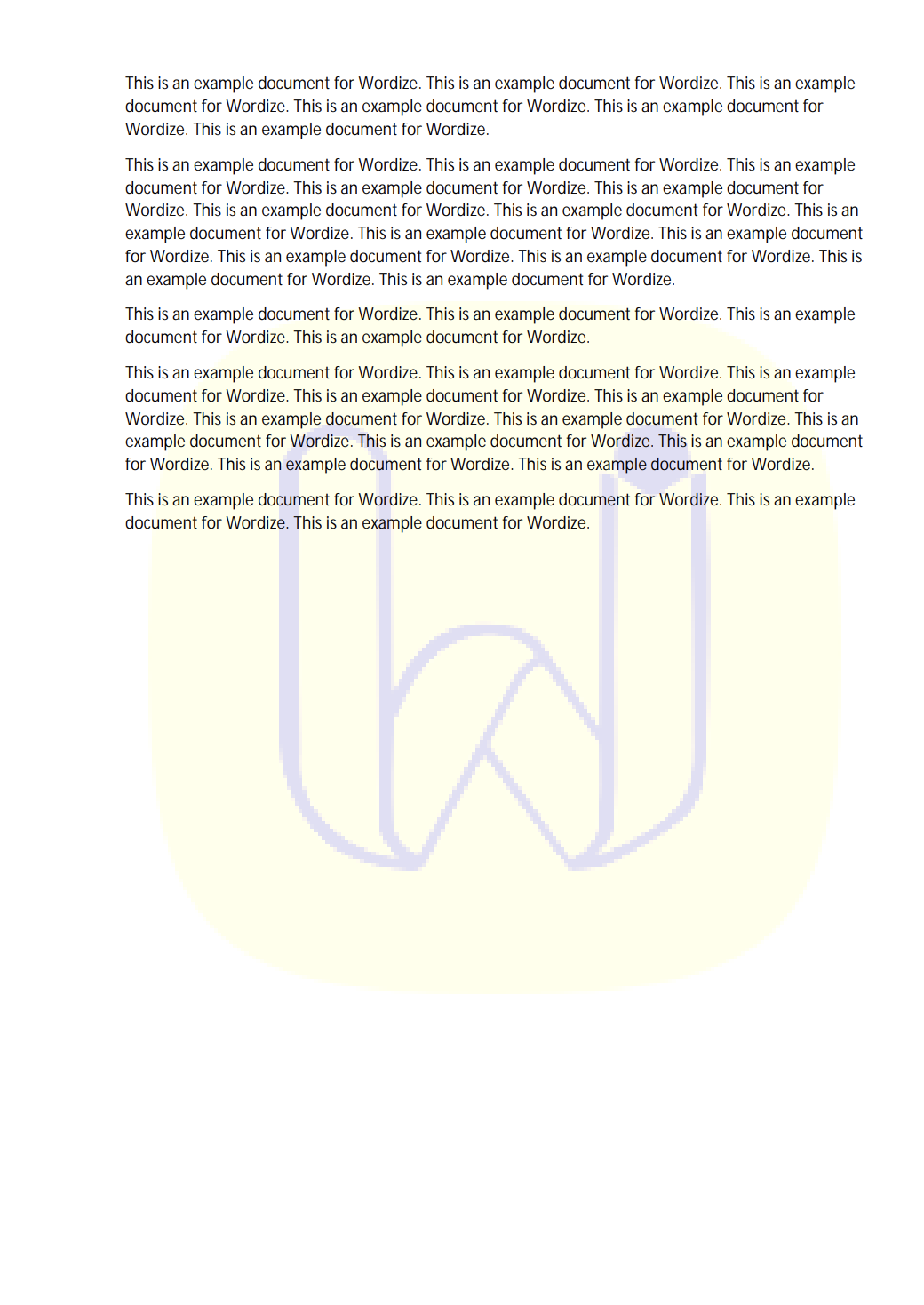
Use one of the SetImage methods to add an image watermark to a document:
method SetImage(string, string, string)
string doc = "Document.docx";
string watermarkImage = "Logo.jpg";
Watermarker.SetImage(doc, "WatermarkImage.1.docx", watermarkImage);
method SetImage(string, string, SaveFormat, string)
string doc = "Document.docx";
string watermarkImage = "Logo.jpg";
Watermarker.SetImage(doc, "WatermarkImage.2.docx", SaveFormat.Docx, watermarkImage);
method SetImage(Stream, Stream, SaveFormat, Image)
using var streamIn = File.OpenRead("Document.docx");
using var streamOut = File.Create("WatermarkImageStream.1.docx");
Watermarker.SetImage(streamIn, streamOut, SaveFormat.Docx, System.Drawing.Image.FromFile("Logo.jpg"));
Image Watermark Options
Wordize allows you to customize the image watermark using ImageWatermarkOptions:
- Scale – to specify the scale factor expressed as a fraction of the image, the default value is 0 - auto, valid values range from 0 to 65.5 inclusive
- IsWashout – specifies a boolean value that is responsible for the washout effect of the watermark, the default value is “true”
The following code examples show how to customize a text watermark using one of the SetImage methods:
method SetImage(string, string, string, ImageWatermarkOptions)
string doc = "Document.docx";
string watermarkImage = "Logo.jpg";
ImageWatermarkOptions options = new ImageWatermarkOptions();
options.Scale = 50;
Watermarker.SetImage(doc, "WatermarkImage.3.docx", watermarkImage, options);
method SetImage(string, string, SaveFormat, string, ImageWatermarkOptions)
string doc = "Document.docx";
string watermarkImage = "Logo.jpg";
ImageWatermarkOptions options = new ImageWatermarkOptions();
options.Scale = 50;
Watermarker.SetImage(doc, "WatermarkImage.4.docx", SaveFormat.Docx, watermarkImage, options);
method ImageWatermarkOptions(Stream, Stream, SaveFormat, Image, ImageWatermarkOptions)
using var streamIn = File.OpenRead("Document.docx");
using var streamOut = File.Create("WatermarkImageStream.2.docx");
Watermarker.SetImage(streamIn, streamOut, SaveFormat.Docx, System.Drawing.Image.FromFile("Logo.jpg"), new ImageWatermarkOptions() { Scale = 50 });
Adding a Text Watermark
The functionality of adding a text watermark in Wordize is available through the Watermarker class, which provides SetText methods.
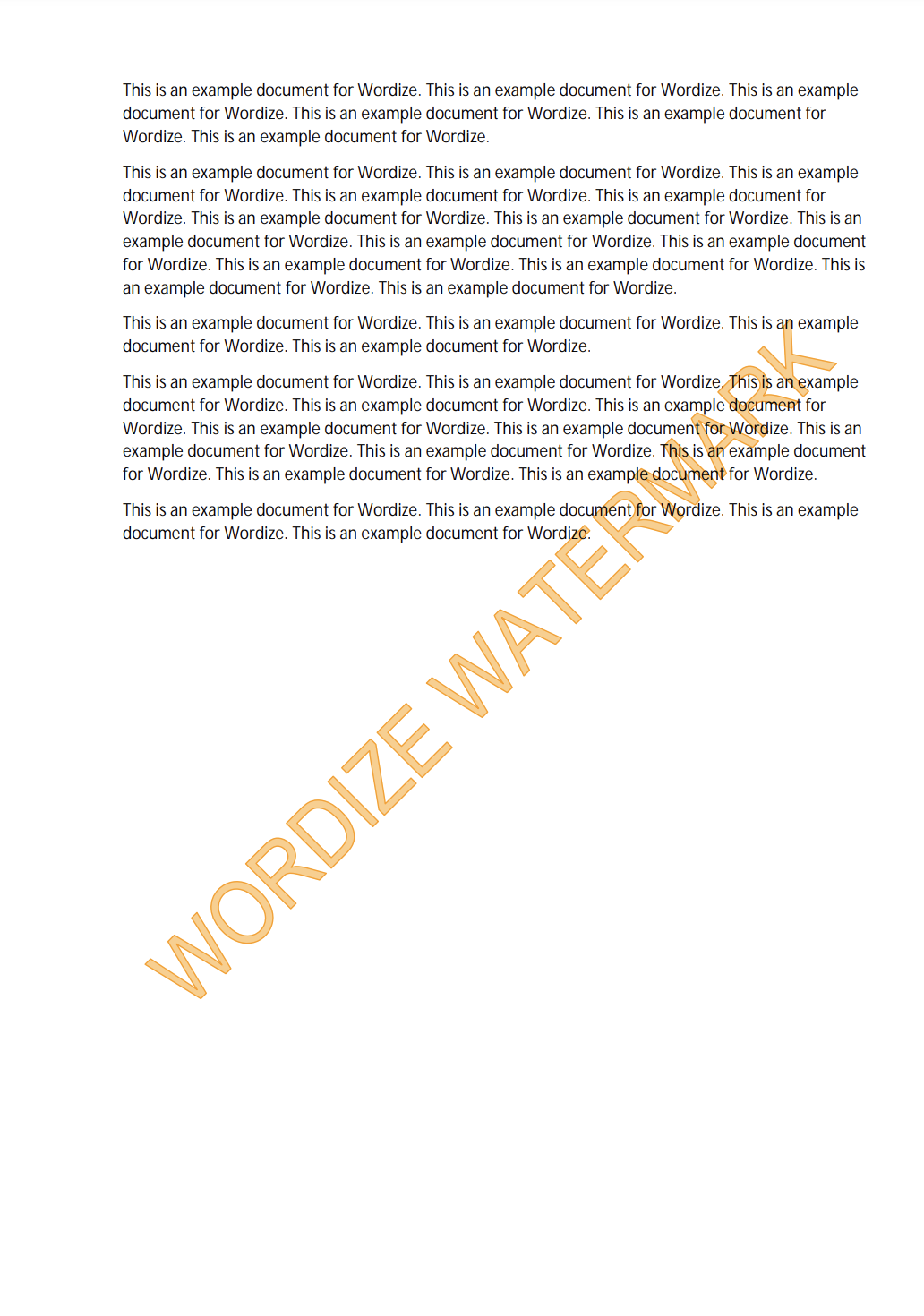
Use one of the SetText methods to add a text watermark to a document:
method SetText(string, string, string)
string doc = "Document.docx";
string watermarkText = "This is a watermark";
Watermarker.SetText(doc, "WatermarkText.1.docx", watermarkText);
method SetText(string, string, SaveFormat, string)
string doc = "Document.docx";
string watermarkText = "This is a watermark";
Watermarker.SetText(doc, "WatermarkText.2.docx", SaveFormat.Docx, watermarkText);
method SetText(Stream, Stream, SaveFormat, string)
using var streamIn = File.OpenRead("Document.docx");
using var streamOut = File.Create("WatermarkTextStream.1.docx");
Watermarker.SetText(streamIn, streamOut, SaveFormat.Docx, watermarkText);
Text Watermark Options
Wordize also allows you to customize the text watermark using TextWatermarkOptions:
- FontFamily – to specify the font family name, the default value is “Calibri”
- Color – to specify the font color, the default value is “Silver”
- FontSize – to specify the font size, the default value is 0 – “auto”
- IsSemitrasparent – to specify a boolean value that controls the watermark opacity, the default value is “true”
- Layout – to specify the watermark layout, the default value is “Diagonal”
The following code examples show how to customize a text watermark using one of the SetText methods:
method SetText(string, string, string, TextWatermarkOptions)
string doc = "Document.docx";
string watermarkText = "This is a watermark";
TextWatermarkOptions watermarkOptions = new TextWatermarkOptions();
watermarkOptions.Color = Color.Red;
Watermarker.SetText(doc, "WatermarkText.3.docx", watermarkText, watermarkOptions);
method SetText(string, string, SaveFormat, string, TextWatermarkOptions)
string doc = "Document.docx";
string watermarkText = "This is a watermark";
TextWatermarkOptions watermarkOptions = new TextWatermarkOptions();
watermarkOptions.Color = Color.Red;
Watermarker.SetText(doc, "WatermarkText.4.docx", SaveFormat.Docx, watermarkText, watermarkOptions);
method SetText(Stream, Stream, SaveFormat, string, TextWatermarkOptions)
using var streamIn = OpenRead("Document.docx");
using var streamOut = File.Create("WatermarkTextStream.2.docx");
TextWatermarkOptions options = new TextWatermarkOptions();
options.Color = Color.Red;
Watermarker.SetText(streamIn, streamOut, SaveFormat.Docx, watermarkText, options);