Search and Replace
Use the Wordize Replacement for .NET module to search and replace some parts of your document.
Select any document conversion module to work with the required formats.
Wordize provides powerful search and replace functionality, allowing developers to find specific text or patterns in documents and replace them programmatically. This feature is useful for updating templates, correcting errors, and automating content modifications across multiple documents.
With Wordize Replacement for .NET, you can:
- Find and replace plain text
- Use regular expressions for advanced search operations
Search and Replace Simple Text
The simplest way to replace text in a document is by providing a search string and a replacement string. This method is useful for making direct word or phrase substitutions.
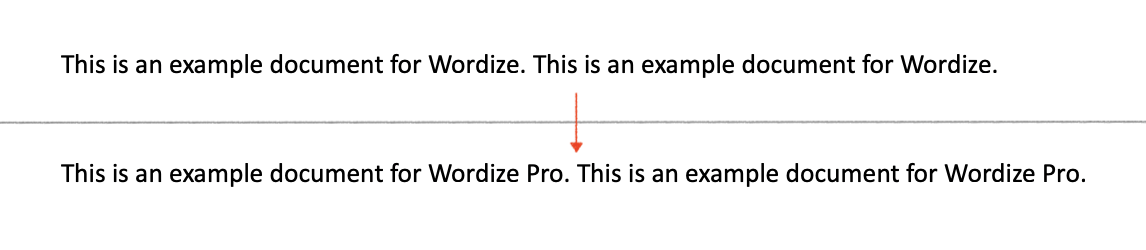
The following code example shows how to perform search and replace on a string:
var doc = "Document.docx";
var pattern = "Wordize";
var replacement = "Wordize Pro";
Replacer.Replace(doc, "Document.1.docx", pattern, replacement);
Find a full list of code examples for search and replace in the Search and Replace Code Examples section at the bottom of the page.
Search and Replace Using Regular Expressions
Wordize provides a powerful way to search and replace documents using regular expressions (Regex). This is useful when working with dynamic text such as dates, numbers, placeholders, or recurring phrases. Using Wordize Replacer for .NET and the Replace method options, developers can define complex search patterns and replace them with the desired text.
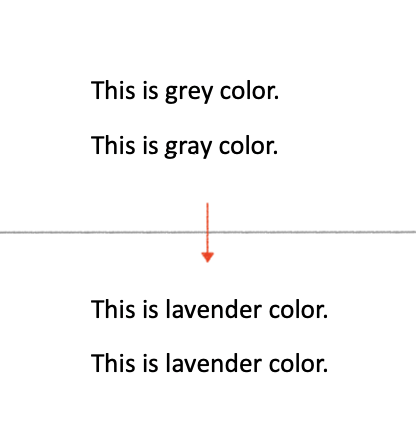
The following code example shows how to perform search and replace through a regular expression:
var doc = "Document.docx";
Regex pattern = new Regex("gr(a|e)y");
var replacement = "lavender";
Replacer.Replace(doc, "Document.4.docx", pattern, replacement);
Find a full list of code examples for search and replace in the Search and Replace Code Examples section at the bottom of the page.
Customize Search and Replace
You can work with many options during the search and replace process using the following FindReplaceOptions:
- ReplacementFormat – to specify the replacement format, defaults to “Text”
- MatchCase – “true” if the search is case sensitive, “false” if the search is case insensitive
- FindWholeWordsOnly – to specify whether we are searching for a standalone word
- IgnoreDeleted – to specify whether to ignore text inside delete revisions, defaults to “false”
- IgnoreInserted – to specify whether to ignore text inside insert revisions, defaults to “false”
- IgnoreFields – to specify whether to ignore text inside fields, defaults to “false”
- IgnoreFieldCodes – to specify whether to ignore field codes only, defaults to “false”
- IgnoreFootnotes – to specify whether to ignore footnotes, defaults to “false”
- UseSubstitutions – to specify whether to recognize and use substitutions within replacement patterns, defaults to “false”
- IgnoreStructuredDocumentTags – to specify whether to ignore the content of SDT, defaults to “false”
- IgnoreShapes – to specify whether to ignore shapes within a text, defaults to “false”
The following code example shows how to perform search and replace with additional options: To perform a search and replace with FindReplaceOptions, use one of the following Replace methods:
var doc = "Document.doc";
var pattern = "Wordize";
var replacement = "Wordize Pro";
FindReplaceOptions options = new FindReplaceOptions { FindWholeWordsOnly = true };
Replacer.Replace(doc, "Document.7.docx", SaveFormat.Docx, pattern, replacement, options);
Find a full list of code examples for search and replace in the Search and Replace Code Examples section at the bottom of the page.
Popular User Scenarios
The following code example shows how to specify ReplacementFormat to HTML:
var doc = "Document.doc";
var pattern = "run";
var replacement = "<b>Text </b><i>with </i><u>formatting</u>";
FindReplaceOptions options = new FindReplaceOptions() { ReplacementFormat = ReplacementFormat.Html };
Replacer.Replace(doc, "Document.7.docx", SaveFormat.Docx, pattern, replacement, options);
The following code example shows how to specify ReplacementFormat to Markdown:
var doc = "Document.doc";
var pattern = "run";
var replacement = "**Text** *with* `formatting`";
FindReplaceOptions options = new FindReplaceOptions() { ReplacementFormat = ReplacementFormat.Markdown };
Replacer.Replace(doc, "Document.8.docx", SaveFormat.Docx, pattern, replacement, options);
The following code example shows how to replace text only if the found pattern is a separate word:
var doc = "Document.doc";
var pattern = "Wordize";
var replacement = "Wordize Pro";
FindReplaceOptions options = new FindReplaceOptions { FindWholeWordsOnly = true };
Replacer.Replace(doc, "Document.9.docx", SaveFormat.Docx, pattern, replacement, options);
The following code example shows how to ignore text inside fields when replacing:
var doc = "Document.doc";
var pattern = "Wordize";
var replacement = "Wordize Pro";
FindReplaceOptions options = new FindReplaceOptions { IgnoreFields = true };
Replacer.Replace(doc, "Document.10.docx", SaveFormat.Docx, pattern, replacement, options);
The following code example shows how to how to replace all occurrences of a specified character string pattern with a replacement string in an input file and save the result to an image:
Stream[] pages = Replacer.ReplaceToImages("Document1.docx", new ImageSaveOptions(SaveFormat.Png), "Wordize", "(Wordize Pro)");
using var streamIn = File.OpenRead("Document1.docx");
Stream[] pages = Replacer.ReplaceToImages(streamIn, new ImageSaveOptions(SaveFormat.Png), "Wordize", "(Wordize Pro)");
Search and Replace Code Examples
Wordize provides both a Standard API and a Fluent API, allowing developers to choose the most convenient approach for their needs.
Wordize API
Search and Replace on a String
method Replace(string, string, string, string)
var doc = "Document.docx";
var pattern = "Wordize";
var replacement = "Wordize Pro";
Replacer.Replace(doc, "Document.1.docx", pattern, replacement);
method Replace(string, string, SaveFormat, string, string)
var doc = "Document.doc";
var pattern = "Wordize";
var replacement = "Wordize Pro";
Replacer.Replace(doc, "Document.2.docx", SaveFormat.Docx, pattern, replacement);
method Replace(Stream, Stream, SaveFormat, string, string)
var pattern = "Wordize";
var replacement = "Wordize Pro";
using var streamIn = File.OpenRead("Document.doc");
using var streamOut = File.Create("Document.3.docx");
Replacer.Replace(streamIn, streamOut, SaveFormat.Docx, pattern, replacement);
Search and Replace Using Regex
method Replace(string, string, Regex, string)
var doc = "Document.docx";
Regex pattern = new Regex("gr(a|e)y");
var replacement = "lavender";
Replacer.Replace(doc, "Document.4.docx", pattern, replacement);
method Replace(string, string, SaveFormat, Regex, string)
var doc = "Document.doc";
Regex pattern = new Regex("gr(a|e)y");
var replacement = "lavender";
Replacer.Replace(doc, "Document.5.docx", SaveFormat.Docx, pattern, replacement);
method Replace(Stream, Stream, SaveFormat, Regex, string)
Regex pattern = new Regex("gr(a|e)y");
var replacement = "lavender";
using var streamIn = File.OpenRead("Document.docx");
using var streamOut = File.Create("Document.6.docx");
Replacer.Replace(streamIn, streamOut, SaveFormat.Docx, pattern, replacement);
Search and Replace Using Additional Options
method Replace(string, string, SaveFormat, string, string, FindReplaceOptions)
var doc = "Document.doc";
var pattern = "Wordize";
var replacement = "Wordize Pro";
FindReplaceOptions options = new FindReplaceOptions { FindWholeWordsOnly = true };
Replacer.Replace(doc, "Document.7.docx", SaveFormat.Docx, pattern, replacement, options);
method Replace(string, string, SaveFormat, Regex, string, FindReplaceOptions)
var doc = "Document.doc";
Regex pattern = new Regex("gr(a|e)y");
var replacement = "lavender";
FindReplaceOptions options = new FindReplaceOptions { FindWholeWordsOnly = true };
Replacer.Replace(doc, "Document.8.docx", SaveFormat.Docx, pattern, replacement, options);
method Replace(Stream, Stream, SaveFormat, String, String, FindReplaceOptions)
var pattern = "Wordize";
var replacement = "Wordize Pro";
using var streamIn = File.OpenRead("Document.doc");
using var streamOut = File.Create("Document.9.docx");
Replacer.Replace(streamIn, streamOut, SaveFormat.Docx, pattern, replacement, new FindReplaceOptions { FindWholeWordsOnly = true });
method Replace(Stream, Stream, SaveFormat, Regex, string, FindReplaceOptions)
Regex pattern = new Regex("gr(a|e)y");
var replacement = "lavender";
using var streamIn = File.OpenRead("Document.doc");
using var streamOut = File.Create("Document.10.docx");
Replacer.Replace(streamIn, streamOut, SaveFormat.Docx, pattern, replacement, new FindReplaceOptions { FindWholeWordsOnly = true });
Wordize Fluent API
Within the Fluent API, there is a context. ReplacerContext allows you to customize the document search and replace process. You can search and replace in documents with or without context.
method to Search and replace a string
ReplacerContext replacerContext = new ReplacerContext();
replacerContext.SetReplacement("ReplaceMe", "Replacement");
Replacer.Create(replacerContext)
.From("DocumentIn.docx")
.To("DocumentOut.docx")
.Execute();
method to Search and replace through a regular expression
ReplacerContext replacerContext = new ReplacerContext();
replacerContext.SetReplacement(new Regex("gr(a|e)y"), "lavender");
Replacer.Create(replacerContext)
.From("DocumentIn.docx")
.To("DocumentOut.docx")
.Execute();
method to Search and replace through a regular expression with markup-formatted text
ReplacerContext replacerContext = new ReplacerContext();
replacerContext.SetReplacement(new Regex(@"oc[a-z]+e"), "**Text** *with* `formatting`");
replacerContext.FindReplaceOptions.ReplacementFormat = ReplacementFormat.Markdown;
Replacer.Create(replacerContext)
.From("DocumentIn.docx")
.To("DocumentOut.docx")
.Execute();