Mail Merge
Use the Wordize Mail Merge for .NET module to automate the creation of personalized documents.
Select any document conversion module to work with the required formats.
Wordize allows users to automate the creation of personalized documents such as letters, invoices, or emails. It works by combining a template with data from sources like spreadsheets or databases, which is called Mail Merge. This saves time, reduces errors, and allows for efficient batch document generation.
How Mail Merge Works
Mail Merge is a process that combines a document template with data to create personalized documents in bulk. It works by inserting specific fields (e.g., names, addresses) into a template, which is then filled with data from a data source, generating multiple customized outputs.
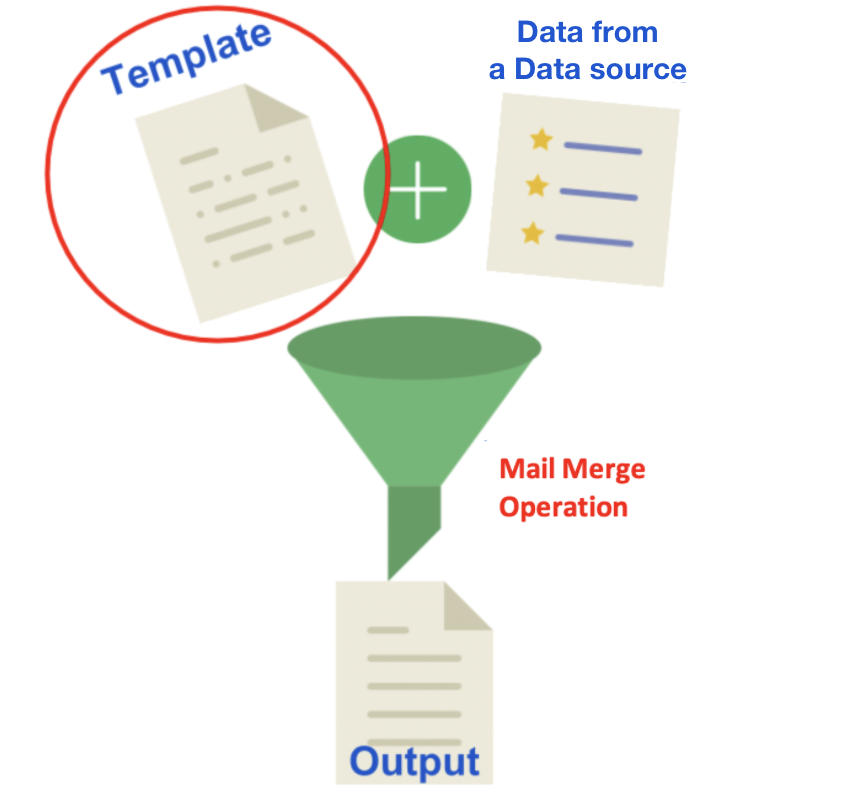
Wordize allows users to use DataRow, DataTable, DataSet, and an array of values as data sources.
Simple Mail Merge Operation
Simple Mail Merge creates personalized documents by merging a template with data. In the template, placeholders (called merge fields) represent dynamic content like names, addresses, or dates. During the merge process, these placeholders are replaced with the corresponding data from an external data source.
You can add one or more merge fields to your template and then run a simple mail merge operation. It is important to understand that the output documents will differ only in the specific values in the merge fields.
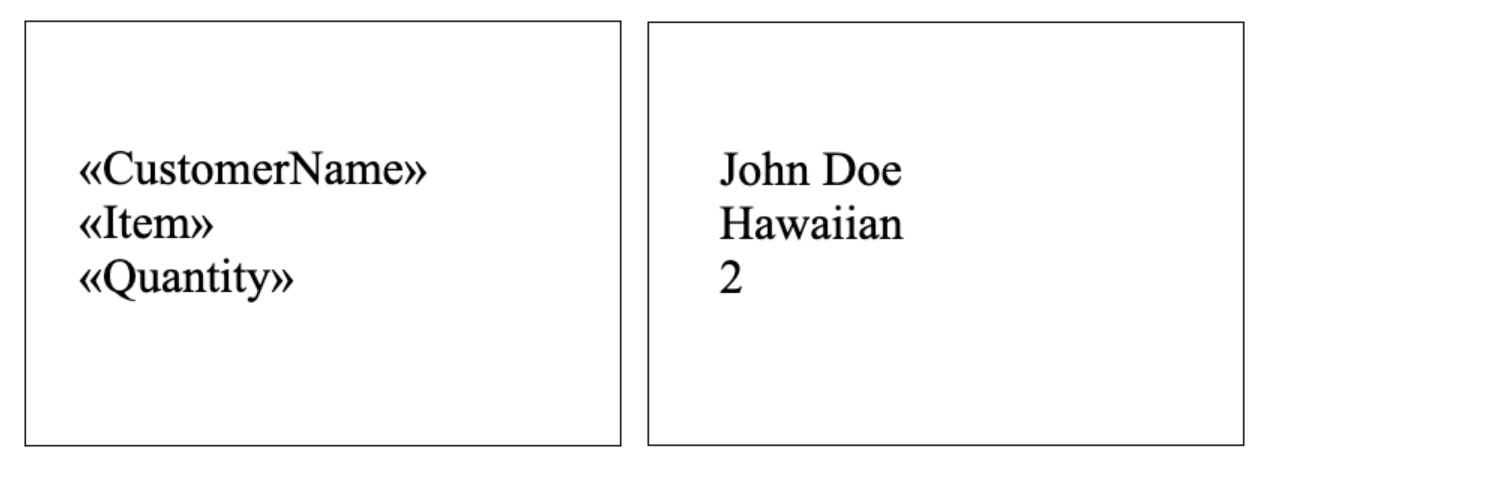
Example workflow:
- Prepare a template document with merge fields (e.g.
<<FirstName>>
,<<Location>>
,<<Quantity>>
) - Prepare data in the data source (DataRow, DataTable, DataSet, array of values)
- Execute the merge operation to produce individual documents with personalized information
Look at the templates for performing the mail merge operation:
Use the Simple template and one of the Execute methods to perform a simple merge operation:
method Execute(string, string, string[], object[])
string doc = "MergeTemplate.docx";
string[] fieldNames = new string[] { "FirstName", "Location", "Quantity" };
string[] fieldValues = new string[] { "James Bond", "London", "7" };
MailMerger.Execute(doc, "MailMerge.1.docx", fieldNames, fieldValues);
method Execute(string, string, SaveFormat, string[], object[])
string doc = "MergeTemplate.docx";
string[] fieldNames = new string[] { "FirstName", "Location", "Quantity" };
string[] fieldValues = new string[] { "James Bond", "London", "7" };
MailMerger.Execute(doc, "MailMerge.2.docx", SaveFormat.Docx, fieldNames, fieldValues);
method Execute(string, string, DataRow)
string doc = "MergeTemplate.docx;
DataTable dataTable = new DataTable();
dataTable.Columns.Add("FirstName");
dataTable.Columns.Add("Location");
dataTable.Columns.Add("Quantity");
DataRow dataRow = dataTable.Rows.Add(new string[] { "James Bond", "London", "7" });
MailMerger.Execute(doc, "MailMergeDataRow.1.docx", dataRow);
method Execute(string, string, SaveFormat, DataRow)
string doc = "MergeTemplate.docx;
DataTable dataTable = new DataTable();
dataTable.Columns.Add("FirstName");
dataTable.Columns.Add("Location");
dataTable.Columns.Add("Quantity");
DataRow dataRow = dataTable.Rows.Add(new string[] { "James Bond", "London", "7" });
MailMerger.Execute(doc, "MailMergeDataRow.2.docx", SaveFormat.Docx, dataRow);
method Execute(string, string, DataTable)
string doc = "MergeTemplate.docx;
DataTable dataTable = new DataTable();
dataTable.Columns.Add("FirstName");
dataTable.Columns.Add("Location");
dataTable.Columns.Add("Quantity");
DataRow dataRow = dataTable.Rows.Add(new string[] { "James Bond", "London", "7" });
MailMerger.Execute(doc, "MailMergeDataTable.1.docx", dataTable);
method Execute(string, string, SaveFormat, DataTable)
string doc = "MergeTemplate.docx;
DataTable dataTable = new DataTable();
dataTable.Columns.Add("FirstName");
dataTable.Columns.Add("Location");
dataTable.Columns.Add("Quantity");
DataRow dataRow = dataTable.Rows.Add(new string[] { "James Bond", "London", "7" });
MailMerger.Execute(doc, "MailMergeDataTable.2.docx", SaveFormat.Docx, dataTable);
method Execute(Stream, Stream, SaveFormat, string[], object[])
string[] fieldNames = new string[] { "FirstName", "Location", "Quantity" };
string[] fieldValues = new string[] { "James Bond", "London", "7" };
using var streamIn = File.OpenRead("MergeTemplate.docx);
using var streamOut = File.Create("MailMergeStream.1.docx");
MailMerger.Execute(streamIn, streamOut, SaveFormat.Docx, fieldNames, fieldValues);
method Execute(Stream, Stream, SaveFormat, DataRow)
DataTable dataTable = new DataTable();
dataTable.Columns.Add("FirstName");
dataTable.Columns.Add("Location");
dataTable.Columns.Add("Quantity");
DataRow dataRow = dataTable.Rows.Add(new string[] { "James Bond", "London", "7" });
using var streamIn = File.OpenRead("MergeTemplate.docx);
using var streamOut = File.Create("MailMergeStreamDataRow.1.docx");
MailMerger.Execute(streamIn, streamOut, SaveFormat.Docx, dataRow);
method Execute(Stream, Stream, SaveFormat, DataTable)
DataTable dataTable = new DataTable();
dataTable.Columns.Add("FirstName");
dataTable.Columns.Add("Location");
dataTable.Columns.Add("Quantity");
DataRow dataRow = dataTable.Rows.Add(new string[] { "James Bond", "London", "7" });
using var streamIn = File.OpenRead("MergeTemplate.docx);
using var streamOut = File.Create("MailMergeDataTable.1.docx");
MailMerger.Execute(streamIn, streamOut, SaveFormat.Docx, dataTable);
Mail Merge with Regions
Mail merge with regions is used when you need to insert dynamic content (such as tables with different numbers of rows or itemized lists of different lengths) for a specific area of the document. In this case, merge regions are used instead of simple merge fields – they define sections that are repeated for each data record. This is useful for documents like invoices with multiple line items or reports with dynamic tables.
You can add one or more merge regions to your template and then perform a mail merge operation with the regions.
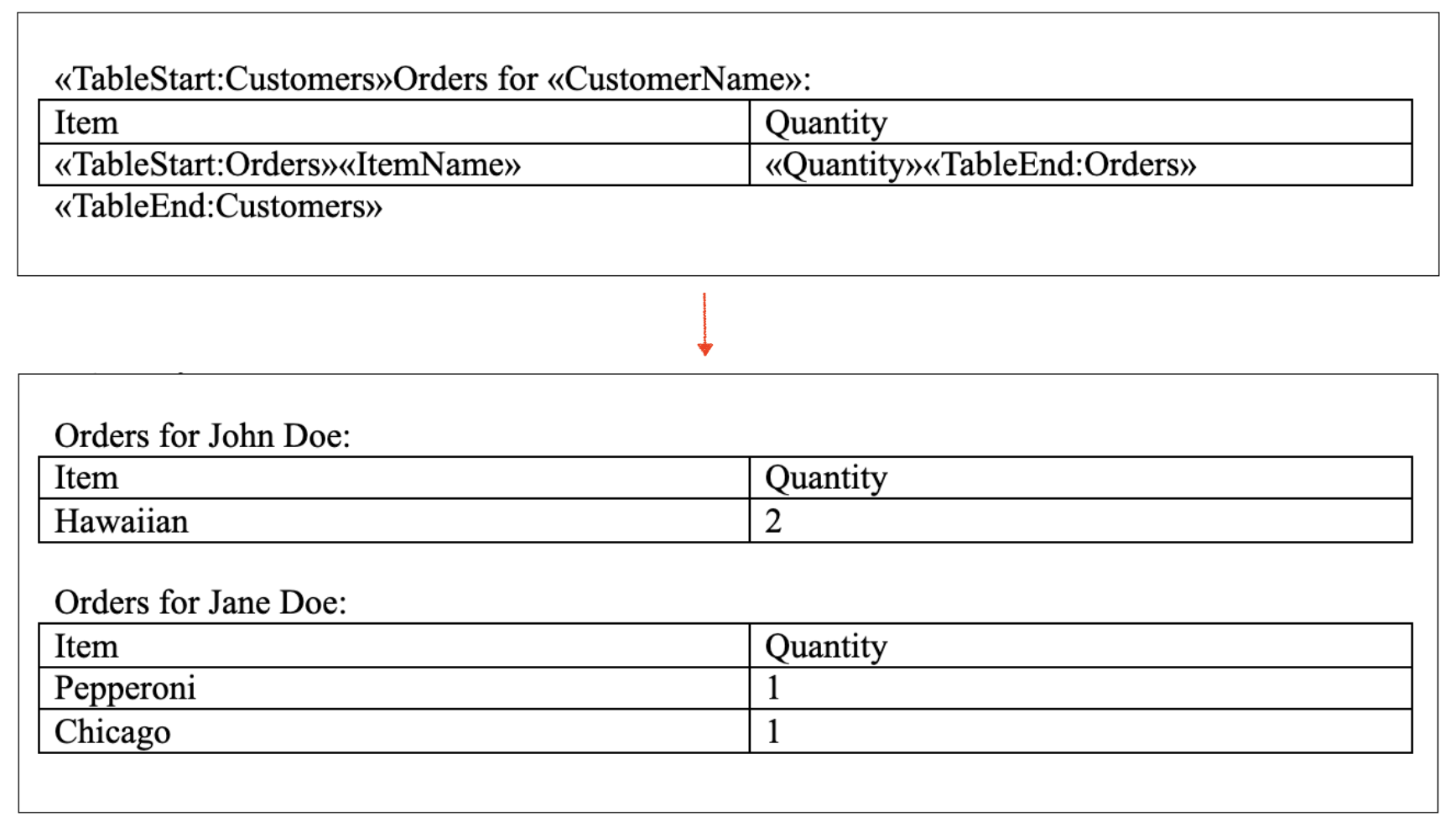
Example workflow:
- Define the merge region in the template using start and end markers (e.g.,
<<TableStart:Items>>
and<<TableEnd:Items>>
) - Connect the template to a structured data source with nested records
- Perform the merge operation
This advanced method provides greater flexibility to handle complex scenarios with multiple data records.
Look at the templates for performing the mail merge with regions operation:
Use the Simple template (for code examples with a DataTable parameter) or the Data Set template template (for code examples with a DataSet parameter) and one of the ExecuteWithRegions methods to perform a mail merge operation with regions:
method ExecuteWithRegions(string, string, DataTable)
string doc = "MergeRegionsTemplate.docx";
DataTable dataTable = new DataTable("MyTable");
dataTable.Columns.Add("FirstName");
dataTable.Columns.Add("LastName");
dataTable.Rows.Add(new object[] { "John", "Doe" });
dataTable.Rows.Add(new object[] { "", "" });
dataTable.Rows.Add(new object[] { "Jane", "Doe" });
MailMerger.ExecuteWithRegions(doc, "MailMergeWithRegionsDataTable.1.docx", dataTable);
method ExecuteWithRegions(string, string, SaveFormat, DataTable)
string doc = "MergeRegionsTemplate.docx";
DataTable dataTable = new DataTable("MyTable");
dataTable.Columns.Add("FirstName");
dataTable.Columns.Add("LastName");
dataTable.Rows.Add(new object[] { "John", "Doe" });
dataTable.Rows.Add(new object[] { "", "" });
dataTable.Rows.Add(new object[] { "Jane", "Doe" });
MailMerger.ExecuteWithRegions(doc, "MailMergeWithRegionsDataTable.2.docx", SaveFormat.Docx, dataTable);
method ExecuteWithRegions(string, string, DataSet)
string doc = "MergeRegionsTemplate.docx";
DataTable tableCustomers = new DataTable("Customers");
tableCustomers.Columns.Add("CustomerID");
tableCustomers.Columns.Add("CustomerName");
tableCustomers.Rows.Add(new object[] { 1, "John Doe" });
tableCustomers.Rows.Add(new object[] { 2, "Jane Doe" });
DataTable tableOrders = new DataTable("Orders");
tableOrders.Columns.Add("CustomerID");
tableOrders.Columns.Add("ItemName");
tableOrders.Columns.Add("Quantity");
tableOrders.Rows.Add(new object[] { 1, "Hawaiian", 2 });
tableOrders.Rows.Add(new object[] { 2, "Pepperoni", 1 });
tableOrders.Rows.Add(new object[] { 2, "Chicago", 1 });
DataSet dataSet = new DataSet();
dataSet.Tables.Add(tableCustomers);
dataSet.Tables.Add(tableOrders);
dataSet.Relations.Add(tableCustomers.Columns["CustomerID"], tableOrders.Columns["CustomerID"]);
MailMerger.ExecuteWithRegions(doc, "MailMergeWithRegionsDataSet.1.docx", dataSet);
method ExecuteWithRegions(string, string, SaveFormat, DataSet)
string doc = "MergeRegionsTemplate.docx";
DataTable tableCustomers = new DataTable("Customers");
tableCustomers.Columns.Add("CustomerID");
tableCustomers.Columns.Add("CustomerName");
tableCustomers.Rows.Add(new object[] { 1, "John Doe" });
tableCustomers.Rows.Add(new object[] { 2, "Jane Doe" });
DataTable tableOrders = new DataTable("Orders");
tableOrders.Columns.Add("CustomerID");
tableOrders.Columns.Add("ItemName");
tableOrders.Columns.Add("Quantity");
tableOrders.Rows.Add(new object[] { 1, "Hawaiian", 2 });
tableOrders.Rows.Add(new object[] { 2, "Pepperoni", 1 });
tableOrders.Rows.Add(new object[] { 2, "Chicago", 1 });
DataSet dataSet = new DataSet();
dataSet.Tables.Add(tableCustomers);
dataSet.Tables.Add(tableOrders);
dataSet.Relations.Add(tableCustomers.Columns["CustomerID"], tableOrders.Columns["CustomerID"]);
MailMerger.ExecuteWithRegions(doc, "MailMergeWithRegionsDataSet.2.docx", SaveFormat.Docx, dataSet);
method ExecuteWithRegions(Stream, Stream, SaveFormat, DataTable)
DataTable dataTable = new DataTable("MyTable");
dataTable.Columns.Add("FirstName");
dataTable.Columns.Add("LastName");
dataTable.Rows.Add(new object[] { "John", "Doe" });
dataTable.Rows.Add(new object[] { "", "" });
dataTable.Rows.Add(new object[] { "Jane", "Doe" });
using var streamIn = File.OpenRead("MergeRegionsTemplate.doc");
using var streamOut = File.Create("MailMergeStreamWithRegionsDataTable.1.docx");
MailMerger.ExecuteWithRegions(streamIn, streamOut, SaveFormat.Docx, dataTable);
method ExecuteWithRegions(Stream, Stream, SaveFormat, DataSet)
DataTable tableCustomers = new DataTable("Customers");
tableCustomers.Columns.Add("CustomerID");
tableCustomers.Columns.Add("CustomerName");
tableCustomers.Rows.Add(new object[] { 1, "John Doe" });
tableCustomers.Rows.Add(new object[] { 2, "Jane Doe" });
DataTable tableOrders = new DataTable("Orders");
tableOrders.Columns.Add("CustomerID");
tableOrders.Columns.Add("ItemName");
tableOrders.Columns.Add("Quantity");
tableOrders.Rows.Add(new object[] { 1, "Hawaiian", 2 });
tableOrders.Rows.Add(new object[] { 2, "Pepperoni", 1 });
tableOrders.Rows.Add(new object[] { 2, "Chicago", 1 });
DataSet dataSet = new DataSet();
dataSet.Tables.Add(tableCustomers);
dataSet.Tables.Add(tableOrders);
dataSet.Relations.Add(tableCustomers.Columns["CustomerID"], tableOrders.Columns["CustomerID"]);
using var streamIn = File.OpenRead("MergeRegionsTemplate.doc");
using var streamOut = File.Create("MailMergeStreamWithRegionsDataTable.1.docx");
MailMerger.ExecuteWithRegions(streamIn, streamOut, SaveFormat.Docx, dataSet);
Mail Merge Options
Mail Merge options allow you to customize the behavior of the mail merge process, controlling how data is processed, formatted, and displayed in the final document. These options provide flexibility for advanced scenarios and fine-tuning output.
Wordize supports the following MailMergeOptions:
- CleanupOptions – specifies what items should be removed during the mail merge
- CleanupParagraphsWithPunctuationMarks – indicate whether paragraphs with punctuation marks are considered as empty and should be removed if the RemoveEmptyParagraphs option is specified
- MergeDuplicateRegions – indicates whether all of the document mail merge regions with the name of a data source should be merged while executing mail merge with regions against the data source or just the first one
- MergeWholeDocument – indicates whether fields in a whole document are updated while executing mail merge with regions
- PreserveUnusedTags – indicates whether the unused tags should be preserved
- RegionEndTag – specifies a mail merge region end tag
- RegionStartTag – specifies a mail merge region start tag
- RestartListsAtEachSection – indicates whether lists are restarted at each section after executing the mail merge
- RetainFirstSectionStart – indicates whether the section start of the first document section and its copies for subsequent data source rows are retained during mail merge or updated according to MS Word behavior
- TrimWhitespaces – indicates whether trailing and leading whitespaces are trimmed from mail merge values
- UnconditionalMergeFieldsAndRegions – indicates whether merge fields and merge regions are merged regardless of the parent IF field’s condition
- UseNonMergeFields – when true, specifies that in addition to MERGEFIELD fields, mail merge is performed into some other types of fields and also into “{{fieldName}}” tags
- UseWholeParagraphAsRegion – indicates whether a whole paragraph with TableStart or TableEnd field or a particular range between TableStart and TableEnd fields should be included in the mail merge region
Use one of the templates above and the Execute or ExecuteWithRegions methods to perform a mail merge operation with the TrimWhitespaces option:
method Execute(string, string, SaveFormat, MailMergeOptions, string[], object[])
string doc = "MergeTemplate.docx;
string[] fieldNames = new string[] { "FirstName", "Location", "Quantity" };
string[] fieldValues = new string[] { "James Bond", "London", "7" };
MailMergeOptions mailMergeOptions = new MailMergeOptions();
mailMergeOptions.TrimWhitespaces = true;
MailMerger.Execute(doc, "MailMerge.3.docx", SaveFormat.Docx, mailMergeOptions, fieldNames, fieldValues);
method Execute(string, string, SaveFormat, MailMergeOptions, DataRow)
string doc = "MergeTemplate.docx;
DataTable dataTable = new DataTable();
dataTable.Columns.Add("FirstName");
dataTable.Columns.Add("Location");
dataTable.Columns.Add("Quantity");
DataRow dataRow = dataTable.Rows.Add(new string[] { "James Bond", "London", "7" });
MailMerger.Execute(doc, "MailMergeDataRow.3.docx", SaveFormat.Docx, new MailMergeOptions() { TrimWhitespaces = true }, dataRow);
method Execute(string, string, SaveFormat, MailMergeOptions, DataTable)
string doc = "MergeTemplate.docx;
DataTable dataTable = new DataTable();
dataTable.Columns.Add("FirstName");
dataTable.Columns.Add("Location");
dataTable.Columns.Add("Quantity");
DataRow dataRow = dataTable.Rows.Add(new string[] { "James Bond", "London", "7" });
MailMerger.Execute(doc, "MailMergeDataTable.3.docx", SaveFormat.Docx, new MailMergeOptions() { TrimWhitespaces = true }, dataTable);
method Execute(Stream, Stream, SaveFormat, MailMergeOptions, string[], object[])
string[] fieldNames = new string[] { "FirstName", "Location", "Quantity" };
string[] fieldValues = new string[] { "James Bond", "London", "7" };
using var streamIn = File.OpenRead("MergeTemplate.docx);
using var streamOut = File.Create("MailMergeStream.2.docx");
MailMergeOptions mailMergeOptions = new MailMergeOptions();
mailMergeOptions.TrimWhitespaces = true;
MailMerger.Execute(streamIn, streamOut, SaveFormat.Docx, mailMergeOptions, fieldNames, fieldValues);
method Execute(Stream, Stream, SaveFormat, MailMergeOptions, DataRow)
DataTable dataTable = new DataTable();
dataTable.Columns.Add("FirstName");
dataTable.Columns.Add("Location");
dataTable.Columns.Add("Quantity");
DataRow dataRow = dataTable.Rows.Add(new string[] { "James Bond", "London", "7" });
using var streamIn = File.OpenRead("MergeTemplate.docx);
using var streamOut = File.Create("MailMergeStreamDataRow.2.docx");
MailMerger.Execute(streamIn, streamOut, SaveFormat.Docx, new MailMergeOptions() { TrimWhitespaces = true }, dataRow);
method Execute(Stream, Stream, SaveFormat, MailMergeOptions, DataTable)
DataTable dataTable = new DataTable();
dataTable.Columns.Add("FirstName");
dataTable.Columns.Add("Location");
dataTable.Columns.Add("Quantity");
DataRow dataRow = dataTable.Rows.Add(new string[] { "James Bond", "London", "7" });
using var streamIn = File.OpenRead("MergeTemplate.docx);
using var streamOut = File.Create("MailMergeDataTable.2.docx");
MailMerger.Execute(streamIn, streamOut, SaveFormat.Docx, new MailMergeOptions() { TrimWhitespaces = true }, dataTable);
method ExecuteWithRegions(string, string, SaveFormat, MailMergeOptions, DataTable)
string doc = "MergeRegionsTemplate.docx";
DataTable dataTable = new DataTable("MyTable");
dataTable.Columns.Add("FirstName");
dataTable.Columns.Add("LastName");
dataTable.Rows.Add(new object[] { "John", "Doe" });
dataTable.Rows.Add(new object[] { "", "" });
dataTable.Rows.Add(new object[] { "Jane", "Doe" });
MailMerger.ExecuteWithRegions(doc, "MailMergeWithRegionsDataTable.3.docx", SaveFormat.Docx, new MailMergeOptions() { TrimWhitespaces = true }, dataTable);
method ExecuteWithRegions(string, string, SaveFormat, MailMergeOptions, DataSet)
string doc = "MergeRegionsTemplate.docx";
DataTable tableCustomers = new DataTable("Customers");
tableCustomers.Columns.Add("CustomerID");
tableCustomers.Columns.Add("CustomerName");
tableCustomers.Rows.Add(new object[] { 1, "John Doe" });
tableCustomers.Rows.Add(new object[] { 2, "Jane Doe" });
DataTable tableOrders = new DataTable("Orders");
tableOrders.Columns.Add("CustomerID");
tableOrders.Columns.Add("ItemName");
tableOrders.Columns.Add("Quantity");
tableOrders.Rows.Add(new object[] { 1, "Hawaiian", 2 });
tableOrders.Rows.Add(new object[] { 2, "Pepperoni", 1 });
tableOrders.Rows.Add(new object[] { 2, "Chicago", 1 });
DataSet dataSet = new DataSet();
dataSet.Tables.Add(tableCustomers);
dataSet.Tables.Add(tableOrders);
dataSet.Relations.Add(tableCustomers.Columns["CustomerID"], tableOrders.Columns["CustomerID"]);
MailMerger.ExecuteWithRegions(doc, "MailMergeWithRegionsDataSet.3.docx", SaveFormat.Docx, new MailMergeOptions() { TrimWhitespaces = true }, dataSet);
method ExecuteWithRegions(Stream, Stream, SaveFormat, MailMergeOptions, DataTable)
DataTable dataTable = new DataTable("MyTable");
dataTable.Columns.Add("FirstName");
dataTable.Columns.Add("LastName");
dataTable.Rows.Add(new object[] { "John", "Doe" });
dataTable.Rows.Add(new object[] { "", "" });
dataTable.Rows.Add(new object[] { "Jane", "Doe" });
using var streamIn = File.OpenRead("MergeRegionsTemplate.doc");
using var streamOut = File.Create("MailMergeStreamWithRegionsDataTable.2.docx");
MailMerger.ExecuteWithRegions(streamIn, streamOut, SaveFormat.Docx, new MailMergeOptions() { TrimWhitespaces = true }, dataTable);
method ExecuteWithRegions(Stream, Stream, SaveFormat, MailMergeOptions, DataSet)
DataTable tableCustomers = new DataTable("Customers");
tableCustomers.Columns.Add("CustomerID");
tableCustomers.Columns.Add("CustomerName");
tableCustomers.Rows.Add(new object[] { 1, "John Doe" });
tableCustomers.Rows.Add(new object[] { 2, "Jane Doe" });
DataTable tableOrders = new DataTable("Orders");
tableOrders.Columns.Add("CustomerID");
tableOrders.Columns.Add("ItemName");
tableOrders.Columns.Add("Quantity");
tableOrders.Rows.Add(new object[] { 1, "Hawaiian", 2 });
tableOrders.Rows.Add(new object[] { 2, "Pepperoni", 1 });
tableOrders.Rows.Add(new object[] { 2, "Chicago", 1 });
DataSet dataSet = new DataSet();
dataSet.Tables.Add(tableCustomers);
dataSet.Tables.Add(tableOrders);
dataSet.Relations.Add(tableCustomers.Columns["CustomerID"], tableOrders.Columns["CustomerID"]);
using var streamIn = File.OpenRead("MergeRegionsTemplate.doc");
using var streamOut = File.Create("MailMergeStreamWithRegionsDataTable.2.docx");
MailMerger.ExecuteWithRegions(streamIn, streamOut, SaveFormat.Docx, new MailMergeOptions() { TrimWhitespaces = true }, dataSet);
Mail Merge Clenup Option
Mail Merge cleanup options are used to control how the document is cleaned after the merge process. They help remove unused fields, empty paragraphs, and other leftover placeholders, ensuring clean output. These options are especially useful when some merge fields may not have corresponding data.
Wordize supports the following MailMergeCleanupOptions:
- None – to specify a default value
- RemoveEmptyParagraphs – to specify whether paragraphs containing mail merge fields with no data should be removed from the document
- RemoveUnusedRegions – to specify whether unused mail merge regions should be removed from the document
- RemoveUnusedFields – to specify whether unused merge fields should be removed from the document
- RemoveContainingFields – to specify whether fields that contain merge fields should be removed from the document if the nested merge fields are removed
- RemoveStaticFields – to specify whether static fields should be removed from the document
- RemoveEmptyTableRows – to specify whether empty rows containing mail merge regions should be removed from the document
- RemoveEmptyTables – to specify whether to remove from the document tables containing mail merge regions that were removed using either the RemoveUnusedRegions or the RemoveEmptyTableRows option
The following code example shows how to use MailMergeCleanupOptions:
string doc = "MergeTemplate.docx;
string[] fieldNames = new string[] { "FirstName", "Location", "Quantity" };
string[] fieldValues = new string[] { "James Bond", "London", "7" };
MailMergeOptions mailMergeOptions = new MailMergeOptions();
mailMergeOptions.MailMergeCleanupOptions = MailMergeCleanupOptions.RemoveEmptyParagraphs;
MailMerger.Execute(doc, "MailMerge.3.docx", SaveFormat.Docx, mailMergeOptions, fieldNames, fieldValues);